Embarking on your journey in the world of programming begins with setting up the tools you need. Python, a versatile and beginner-friendly programming language, offers a seamless installation process for both beginners and experienced programmers. In this comprehensive guide to Python installation and getting started, we’ll walk you through the steps of installing Python, writing your first program, and understanding the basics of Python syntax.
Quick Python Setup
Before you can start coding in Python, you need to install it on your computer. Follow these steps:
- Visit the Official Python Website: Go to the Python official website and navigate to the “Downloads” section.
- Choose Your Version: Python comes in different versions. As a beginner, select the latest stable version. Click on the download link that matches your operating system (Windows, macOS, or Linux).
- Run the Installer: Once the download is complete, run the installer. Make sure to check the box that says “Add Python to PATH” during installation. This will allow you to run Python from the command line.
- Verify Installation: After installation, open a command prompt (Windows) or terminal (macOS, Linux) and type
python --version
. If the version number is displayed, Python is successfully installed.
Python Installation
Python Syntax: A Fundamental Overview
At the heart of every programming language lies a set of rules and conventions that dictate how code should be written, interpreted, and executed. These rules are collectively known as syntax. Python, a versatile and widely used programming language, has a syntax designed to be both human-readable and efficient. In this article, we’ll delve into the intricacies of Python syntax, exploring its key components, rules, and best practices.
Understanding the Basics
1. Indentation and Code Blocks
One of the most distinctive features of Python’s syntax is its reliance on indentation to define code blocks. Unlike many other programming languages that use braces {}
to group statements, Python uses consistent indentation to delineate blocks of code. This indentation-based approach enforces clean and organized code, making it easier to read and understand.
For example, in a for
loop, you might see:
for i in range(5):
print(i)
print("Inside the loop")
print("Outside the loop")
PythonNotice how the code within the loop is indented, and the consistent indentation indicates which lines of code belong to the loop.
2. Variables and Data Types
In Python, you use variables to store and manage data. A variable is a symbolic name that represents a value. Variables can hold different types of data, such as integers, floating-point numbers, strings, and more. To declare a variable and assign a value to it, you simply use the assignment operator (=
):
name = "Alice"
age = 30
temperature = 98.6
Python3. Statements and Expressions
Python code is composed of statements and expressions. A statement is a complete instruction that performs a specific action, such as assigning a value to a variable or printing a message. An expression, on the other hand, is a combination of variables, operators, and values that evaluates to a single value.
For example, consider the following statement and expression:
Statement:
print("Hello, World!")
PythonWriting Your First Python Program
Now that Python is installed, it’s time to write your first program. The classic “Hello, World!” program is a great starting point.
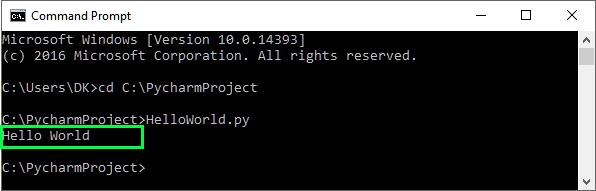
- Open a Text Editor: Open a text editor such as Notepad (Windows), TextEdit (macOS), or any code editor of your choice.
- Write the Code: Type the following code:
- print(“Hello, World!”)
- Save the File: Save the file with an
.py
extension. For example, you can name ithello.py
. - Run the Program: Open a command prompt or terminal, navigate to the directory where you saved the file and type
python hello.py
. You should see the output:Hello, World!
- Understanding Python Syntax: Python syntax refers to the rules that dictate how Python code should be written. Here are a few key points to understand:
- Indentation: Python uses indentation to indicate code blocks. Consistent indentation is crucial for the code to run correctly.
- Comments: Comments are explanatory notes that are not executed by the Python interpreter. They start with the
#
symbol.
# This is a comment
PythonExploring Python Interactive Mode
Python also offers an interactive mode, where you can experiment with code snippets without creating a file. Open a command prompt or terminal and type python
. You’ll enter the interactive mode, denoted by the >>>
prompt. Try typing simple commands like calculations or printing strings, and see immediate results.
External Resources
As you dive deeper into Python, consider exploring these external resources for further learning:
- Python.org: The official Python website offers comprehensive documentation, tutorials, and guides for learners of all levels.
- Codecademy: Codecademy offers interactive Python courses that guide you through the basics and beyond.
Relative Post:
Conclusion
Congratulations! You’ve successfully installed Python and written your first program. With this guide, you’ve taken the first steps into the fascinating world of programming. As you continue your Python journey, remember that practice is key. Experiment with code, explore resources and don’t hesitate to ask questions. With dedication and curiosity, you’ll become a proficient Python programmer in no time.